In Chapter 12 of Learning Perl, we have an exercise for people to practice using the file test operators. Our answer, which can only use the stuff we’ve covered in the book to that point, is simple:
foreach my $file (@ARGV) { my $attribs = &attributes($file); print "'$file' $attribs.\n"; } sub attributes { my $file = shift @_; return "does not exist" unless -e $file; my @attrib; push @attrib, "readable" if -r $file; push @attrib, "writable" if -w $file; push @attrib, "executable" if -x $file; return "exists" unless @attrib; 'is ' . join " and ", @attrib; }
But I usually then redo this to show the same problem creating an Excel file with the same data. Most of you probably already know that the Universe runs on Excel. If you can programmatically create Excel files, you’re half way to controlling the Universe.
This still uses only what we cover in Learning Perl since we have a chapter on using modules, but there’s still a lot of fancy things I’m doing in there that I wouldn’t expect from someone in their first week with Perl.
Now the output is manager and executive friendly:
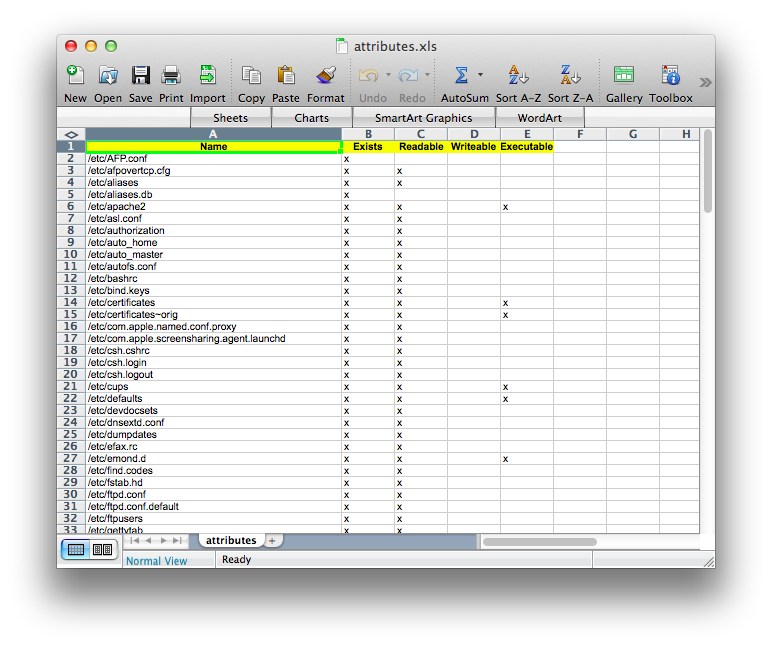
Isn’t there a use statement missing? I mean, which Excel writer module are you talking about?
There’s no use, but there’s a require. It’s hidden in the function that returns the workbook since that’s the only part of the program that needs to know which module to use. Spreadsheet::WriteExcel has the same interface, so I could swap out the module I use for that one.
Small correction to line 10:
Instead of attributes.xls write attributes.xlsx. Then you will be able to open the file in LibreOffice.
I’ve fixed that in the gist. Thanks!
Why do you use
?
I think you’re asking about the
&
at the front of the name. In Learning Perl, we start by telling people to use the&
until they get used to the names of the built-ins. That way, readers know they are always using their version. This is a typical problem in people starting a language, and one of the reasons that Perl has sigils.By the time we use the original example, we’re still putting the
&
out front, so I preserved it here.Could you suggest how to read an excel file which has microarray data? Preferable some sources where I could learn how to do programming in Perl.